So over the past few years I've been working on and off on modules for a game like PERP in Unity3D/C#.
I kept off from creating a post for this as I was unsure where the project was going to be heading, but over the past few weeks I've actually made a few strides in combining these modules into something that is starting to represent PERP more with every update.
If you're interested in following progress, I'll be dropping screenshots here every now and then as stuff actually becomes visual / interactable. These updates are also posted to a Discord dedicated to the project: https://discord.gg/AdACWjmsQc
As it stands, it's mostly just code that is being done, with @Sneaky coming on board to port the Paralake Unreal Engine 4 map to Unity. Any help from people with modelling skills is welcome.
To help out with coding, you'll have to wait a bit since I want the framework to be fully refactored before I let people loose on it, otherwise you'd be wasting time with code that'll need to be changed later anyways, and in turn creating technical debt.
Some really basic screenshots
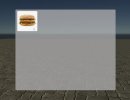
A simple development UI for the inventory
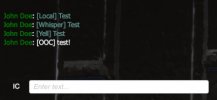
Chatting basically works identical to PERP's chat system, and has multiple channels that can easily be added by implementing a class, which contains checks the server executes to check e.g. wether you're a government employee or in range for local chat.
Some very early development videos (using assets for testing)
I kept off from creating a post for this as I was unsure where the project was going to be heading, but over the past few weeks I've actually made a few strides in combining these modules into something that is starting to represent PERP more with every update.
If you're interested in following progress, I'll be dropping screenshots here every now and then as stuff actually becomes visual / interactable. These updates are also posted to a Discord dedicated to the project: https://discord.gg/AdACWjmsQc
As it stands, it's mostly just code that is being done, with @Sneaky coming on board to port the Paralake Unreal Engine 4 map to Unity. Any help from people with modelling skills is welcome.
To help out with coding, you'll have to wait a bit since I want the framework to be fully refactored before I let people loose on it, otherwise you'd be wasting time with code that'll need to be changed later anyways, and in turn creating technical debt.
Some really basic screenshots
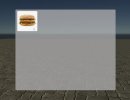
A simple development UI for the inventory
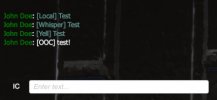
Chatting basically works identical to PERP's chat system, and has multiple channels that can easily be added by implementing a class, which contains checks the server executes to check e.g. wether you're a government employee or in range for local chat.
Some very early development videos (using assets for testing)